- Control Statements in Java is one of the fundamentals required for Java Programming. It allows the smooth flow of a program.
- Java compiler executes the code from top to bottom.
- The statements in the code are executed according to the order in which they appear.
- However, Java provides statements that can be used to control the flow of Java code.
- Such statements are called control flow statements.
Different types of Control Statements:
- Decision Making Statements:
- if statements
- switch statement
- Loop Statements:
- do while loop
- while loop
- for loop
- for-each loop
- Jump Statements:
- break statement
- continue statement
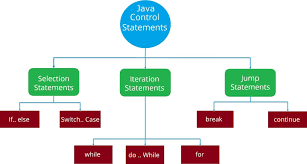
Decision-Making statements:
- Decision-making statements decide which statement to execute and when.
- Decision-making statements evaluate the Boolean expression and control the program flow depending upon the result of the condition provided.
- There are two types of decision-making statements in Java, i.e., If statement and switch statement.

1) If Statement:
- In Java, the “if” statement is used to evaluate a condition.
- The control of the program is diverted depending upon the specific condition.
- The condition of the If statement gives a Boolean value, either true or false.
- In Java, there are four types of if-statements given below.

public class IfExample {
public static void main(String[] args) {
//defining an 'age' variable
int age=20;
//checking the age
if(age>18){
System.out.print("Age is greater than 18");
}
}
}
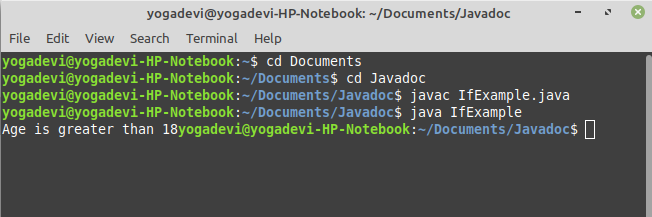
2) if-else statement
- The if -else statement is an extension to the if-statement, which uses another block of code, i.e., else block.
- The else block is executed if the condition of the if-block is evaluated as false.
- The if -else statement is an extension to the if-statement, which uses another block of code, i.e., else block.
- The else block is executed if the condition of the if-block is evaluated as false.
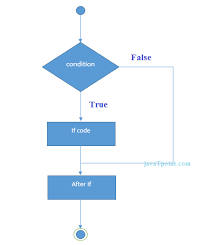
public class Student {
public static void main(String[] args) {
int x = 10;
int y = 12;
if(x+y < 10)
{
System.out.println("x + y is less than 10");
}
else
{
System.out.println("x + y is greater than 20");
}
}
}

3) if-else-if ladder:
- The if-else-if statement contains the if-statement followed by multiple else-if statements.
- In other words, we can say that it is the chain of if-else statements that create a decision tree where the program may enter in the block of code where the condition is true.
- We can also define an else statement at the end of the chain.
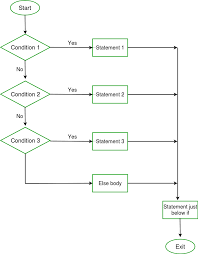
public class Student
{
public static void main(String[] args)
{
String city = "Pudukkottai";
if(city == "Madurai")
{
System.out.println("City is Madurai");
}
else if (city == "Trichy")
{
System.out.println("City is Trichy");
}
else if(city == "Karaikudi")
{
System.out.println("City is Karaikudi");
}
else
{
System.out.println(city);
}
}
}

4. Nested if-statement
In nested if-statements, the if statement can contain a if or if-else statement inside another if or else-if statement.

public class JavaNestedIfExample
{
public static void main(String[] args)
{ //Creating two variables for age and weight
int age=20;
int weight=80; //applying condition on age and weight
if(age>=18)
{
if(weight>50)
{
System.out.println("You are eligible to donate blood");
}
}
}
}
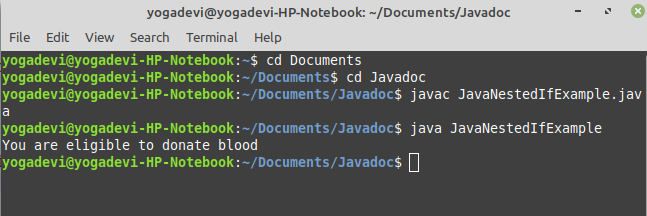
Reference:
https://www.javatpoint.com/control-flow-in-java
https://www.geeksforgeeks.org/decision-making-javaif-else-switch-break-continue-jump/
Leave a comment